Javascript - Review & Loops
Leon Noel
"I ain't know nothin' 'bout no Visa, I was in the park with the gang"

#100Devs
Agenda
- Questions?
- Let's Talk - Next Week
- Review - Pseudo Code
- Review - Variables
- Review - Conditionals
- Review - Functions
- Learn - Loops
- Homework - Work During Break
Questions
About last class or life

Checking In
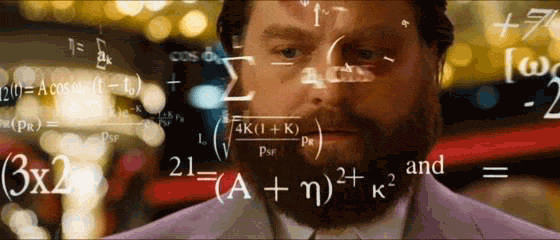
Like and Retweet the Tweet
!checkin
Submitting Work
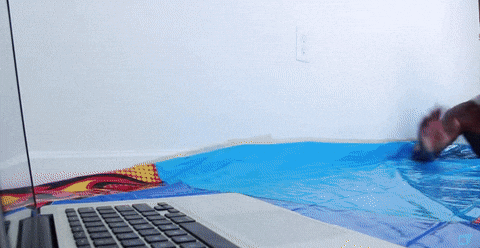
Share What JS You Added in codepen.io
Submit URLs here: https://forms.gle/G7LhHnyTA7zYq7UV6
Thinking by Walter D Wintle
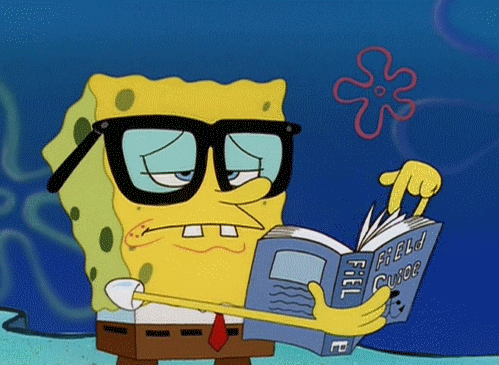
Javascript Should NOT Make Sense YET
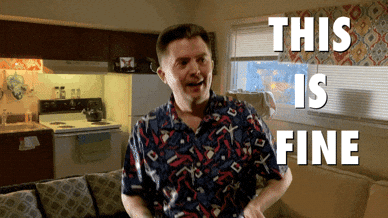
You Should Still Struggle With CSS
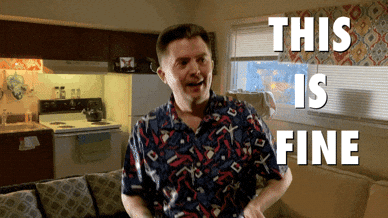
You Should Still Stumble Choosing HTML Tags
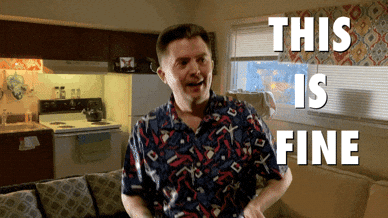
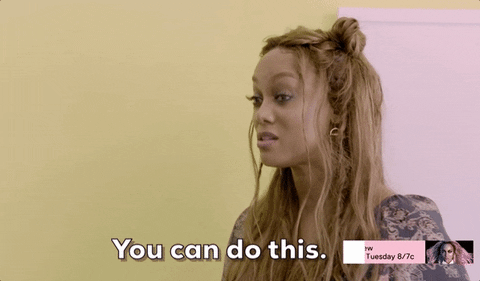
THIS IS ALL NORMAL
YOU CAN AND WILL DO THIS
But What About Freelancing?
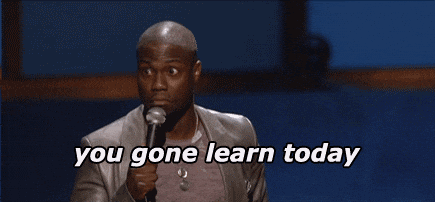
I'm NOT GOING TO
TEXT YOU, CALL YOU, EMAIL YOU OUTSIDE OF THE NEWSLETTER, DM YOU ON TWITTER OR DISCORD, Or EVER ASK YOU FOR MONEY!
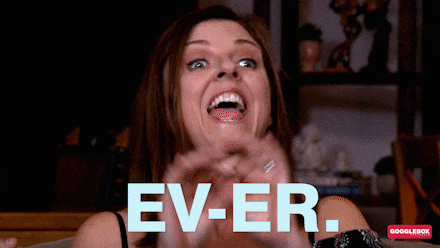
There Are No Other 100Devs Classes or Teachers
But We Have An Amazing Stream Team
https://www.twitch.tv/thedabolical
https://www.twitch.tv/oldcoderchick
And Amazing Folx on Discord
Shout Out @Mayanwolfe
30+ Alumni Mentors!
(yet)
Stream Team Assemble
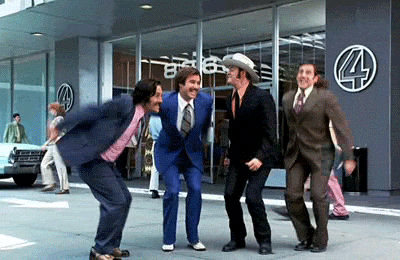
Please Be Kind
Want to be fancy?
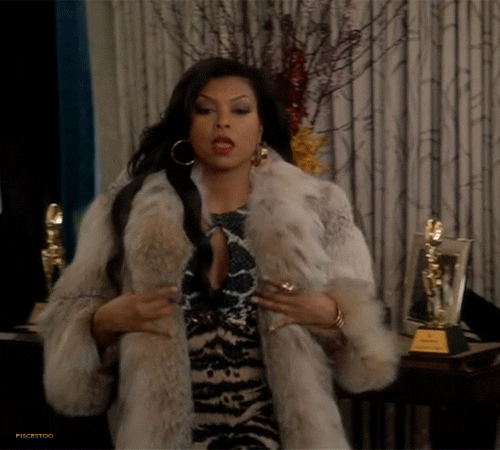
Best Clip Get's Special Color On Discord!
The Winner Is:
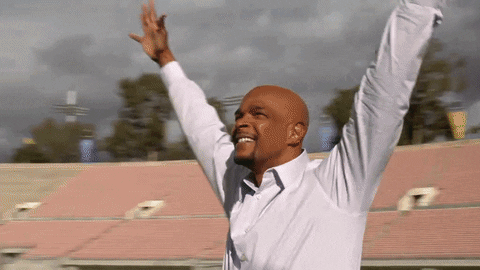
What's Next?
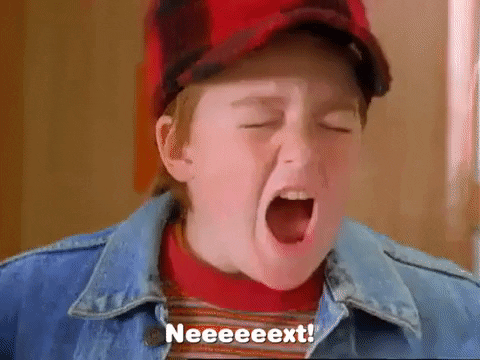
The Golden Rule
SEPERATION OF CONCERNS
- HTML = Content / Structure
- CSS = Style
- JS = Behavior / Interaction
IDs & Classes

IDs
#zebra {
color: red;
}
IDs are used for selecting distinct elements
Only one id with the same value per document
#idName
<section>
<p>Hello, Twitch!</p>
<p id="zebra">Hello, Youtube!</p>
</section>
Classes
.bob {
color: red;
}
Classes are for selecting multiple elements
Multiple with same value allowed per document
.className
<section>
<p class="robot">Hello, Twitch!</p>
<p id="zebra" class="bob">Hello, Youtube!</p>
<p class="bob">Goodbye, Mixer!</p>
</section>
Programming
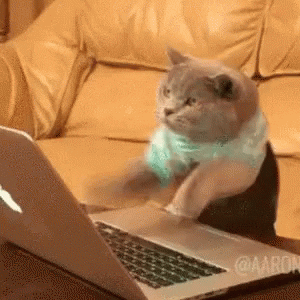
A computer will do what you tell it to do.
What is a program?
A program is a set of instructions that you write to tell a computer what to do
What is a programming?
Programming is the task of writing those instructions in a language that the computer can understand.
True Story
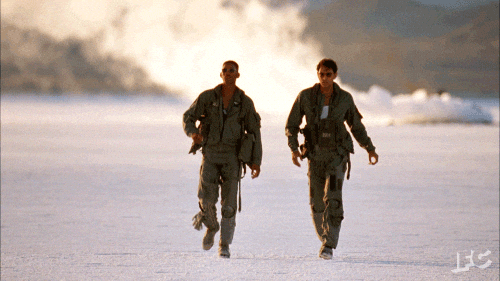
JAVASCRIPT

Has a specific 'Syntax'
Syntax: "Spelling and grammar" rules of a programming language.
Variables
What are variables?
Variables
- We can tell our program to remember values for us to use later on
- The entity we use to store the value is called a variable
Variables
Declaration: let age
Assignment: age = 25
Both at the same time:
let age = 25
Variable Conventions
camelCase:
let numberOfApples = 9
Variables
& Data Types
What can you store in variables?
Strings
- Stores text
- String is surrounded by quotes
"How is the weather today?"
'Warm'
Strings
Double vs Single Quoted Strings:
'They "purchased" it'
"It's a beautiful day"
Numbers
Represent Numerical Data
int: 29
float: 5.14876
Numbers
Signed
int: +4
float: -10.375
Arithmetic In Javascript

Let's Code
Variable Fun Time

Making Decisions
It's either TRUE or FALSE
If you are greater than 18 you are an adult
if (age > 18){
console.log("You are an adult")
}
Comparisons:
Equality
Are two things equal?
9 === 9 //true
7 === 3 //false
"Hello" === "Hello" //true
Logical Operators

Conditional Syntax
if(condition is true) {
//Do cool stuff
}
Conditional Syntax
if(condition is true) {
//Do this cool stuff
}else if(condition is true){
//Do this other cool stuff
}else{
//Default cool stuff
}
Conditional Syntax
const pizza = "Dominos"
if (pizza === "Papa Johns") {
console.log("Scram!")
} else if(pizza === "Dominos") {
console.log("All aboard the train to flavor town")
} else {
console.log("What are you even doing with your life?")
}
🚨 DANGER 🚨
Assignment vs. Comparison
Multiple Conditions
if (name === "Leon" && status === "Ballin"){
//Wink at camera
}
Multiple Conditions
if (day === "Saturday" || day === "Sunday"){
//It is the weekend
}
Let's Code
Age Checker

In Chat:
What is the best TV show of all time?
Answer:
The Bachelor
Functions
What are functions?
Functions
- Functions are simple sets of instructions!
- Functions are reusable
- Functions perform one action as a best practice
- They form the basic "building blocks" of a program
Functions
function name(parameters){
//body
}
//call
name(arguments)
Functions
function yell(word){
alert(word)
}
yell("HELLO")
Functions
function yell(word, otherWord){
alert(word)
alert(otherWord)
}
yell("HELLO","GOODBYE")
Let's Code
Simple Functions

Loops
What are loops?
Loops
- Repeat an action some number of times!
- Three main types of loops in Javascript
- For, while, and do while loops
- Each type offers a different way to determine the start and end points of a loop
Loops - For
for ([initialExpression]; [conditionExpression]; [incrementExpression]){
//do stuff
}
Loops - For
for (let i = 1; i < 5; i++) {
console.log(i)
}
Let's Code
21 Savage Loop

Loops - While
let count = 0
while(count < 5){
console.log(count)
count++
}
Let's Code
Stop Night Snacking

Let's Code
Bring It On!

Let's Code
Bring It On Again!

Homework
Text
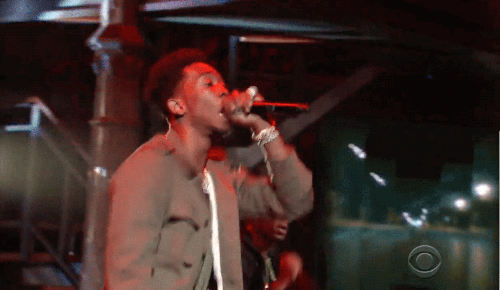
On Discord, you have more than a week!
Office Hours - Sunday 1pm ET